Flipbook is a jQuery plugin that produces a movie effect by running images in quick succession. The concept is similar to something I always did in middle school: doodling in the margins of my notebooks to make short movies. Times have changed and nowadays I use a Javascript object, pulling the images dynamically with jQuery’s AJAX function and a PHP script ;). But don’t worry, it’s just as easy to use as a real flipbook.
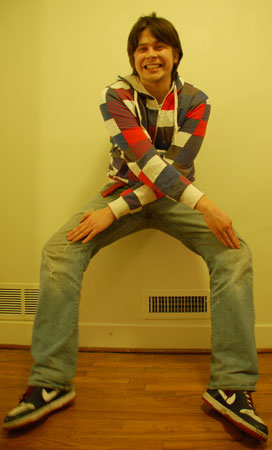
Watch it with Scott Joplin ragtime music.
The jQuery Flipbook Plugin is built around my jQuery slideshow tutorial. It’s very flexible, providing great options for both new and seasoned developers alike. You can easily make anything from a short film to an image slideshow to a text viewer.
Setting up Flipbook
Implementing Flipbook on your website is a piece of cake. First include the necessary Flipbook Javascript and CSS files (be sure to adjust these paths as needed):
<script src="jquery-1.2.6.min.js" type="text/javascript"></script>
<script src="flipbook.js" type="text/javascript"></script>
<link href="flipbook.css" rel="stylesheet" type="text/css" />
It is best to include these in the head of your document, but the upper part of the body should work fine as well. You can leave the Javascript alone, but we will need to make a small modification to the CSS file. Find the blocks #flipbook
and #flipbook IMG
within flipbook.css, and adjust the width and height to match your particular slideshow. For more detailed notes on the CSS see below.
Next we need to add the Flipbook HTML. Flipbook provides a couple of options for how to pull in the images—we can either specify a directory from where Flipbook will grab the images dynamically, provide an array of image paths, or simply place the images (or divs or paragraphs etc.) directly on the page.
Pulling the images from a directory with AJAX
In my opinion, pulling the images from a directory is easiest. To do so, first include this bit of code:
<script type="text/javascript">
$(function() {
Flipbook.ajaxPhp = 'flipbook.php';
Flipbook.init( '/images/flipbook' );
});
</script>
<div id="flipbook">
Loading ...<br />
<a href="http://jonraasch.com/blog/jquery-flipbook-movies">
jQuery Flipbook</a>
</div>
Here we are using the jQuery ready function to initiate the Flipbook once the entire page has loaded. This will pull all the images in alphabetical order from the specified directory and replace the loading content in the flipbook div.
Notice that we set the variable Flipbook.ajaxPhp
. This represents the location of the flipbook.php script, relative to whichever page you are on from the browser’s perspective. Since Javascript runs at the browser level, we have to use AJAX and a PHP script if we want to fetch the directory structure dynamically. Therefore you must upload flipbook.php and adjust Flipbook.ajaxPhp
if you pull the images from a directory. For more advanced use of this PHP script see below.
Pulling the images using an array
However, if you don’t want to bother with the AJAX and PHP scripts, you can call the images manually using an array:
<script type="text/javascript">
$(function() {
Flipbook.init( ['image-1.jpg', 'image-2.jpg', 'images/image-3.jpg' ] );
});
</script>
<div id="flipbook">
Loading ...<br />
<a href="http://jonraasch.com/blog/jquery-flipbook-movies">jQuery Flipbook</a>
</div>
This array stores the paths relative to whichever page you are on in the browser. You can also pass an array of directories, or a combination of directories and image paths if you need to (but then you would need the AJAX/PHP again).
Placing the images (or other markup) directly on the page
Finally, and perhaps easiest for beginners, is including a number of images directly on the page:
<script type="text/javascript">
$(function() {
Flipbook.init();
});
</script>
<div id="flipbook">
<img src="image-1.jpg" />
<img src="image-2.jpg" />
<img src="image-3.jpg" />
</div>
Notice that we passed nothing into the Flipbook.init()
function; this is to prevent the Flipbook script from replacing the content of the flipbook div.
Additionally, flipbook’s versatility allows you to use divs, paragraphs, or any other piece of HTML markup instead of images here. Just make sure to adjust the CSS as necessary.
Customizing Flipbook’s frame rate
Customizing Flipbook couldn’t be easier. To set the frame rate (the speed at which it flips between images), simply set the Flipbook.frameRate
variable before you call Flipbook.init()
:
Flipbook.frameRate = 24;
This would run 24 images every second, or the same quality level as cinematic movies and normal Flash movies. The script does well at handling 12-24 frames per second (FPS), which is very high quality for a flipbook movie running in Javascript. Although the script has been optimized pretty well, I would never run more than 24 FPS to avoid lag on older computers (in fact the default of 8 is perfect for most flipbook-style movies).
Creative customizers can also use the frame rate to change the flipbook into a slideshow. For instance, set Flipbook.frameRate
to 0.2. This will change the slide every 5 seconds. It’s simple mathematics; since the frame rate is calculated by dividing a second by the number of frames, 1 second divided by 0.2 is 5.
Flipbook’s optional fade effect
In addition to the frame rate, the Flipbook plugin for jQuery allows you to implement a fade effect between the images as they flip. Just adjust Flipbook.fadeSpeed
:
Flipbook.fadeSpeed = 100;
This effect works best on slower flipbooks and slideshows. By default the fade speed is set to 0, which allows Flipbook to run better with the faster frame rates. But it’s no loss, since at these higher speeds, the fade tends to detract visually, making the animation look muddy.
Customizing the PHP/AJAX directory listing
Flipbook’s PHP directory listing script allows you to customize a few things. By default, Flipbook’s directory listings are not recursive, meaning it searches a folder, but not any of it’s subfolders. If you would like to make the directory search recursive instead, simply uncomment line 35 in flipbook.php.
Additionally, it’s a good idea to limit the directory listing’s scope for security reasons. Although a hacker can’t necessarily do anything with only the knowledge of your directory structure, it’s very easy to block this script from spidering your entire backend. If you know that you will only want to pull images from a certain directory, modify the commented out preg_match()
on line 21 appropriately.
Advanced CSS info
Developers versed in CSS can customize the Flipbook directly from the stylesheets.
The main CSS concept in these stylesheets is that we are using absolute positioning to stack all the images (or divs, etc.) within the flipbook div. Therefore we have to define absolute positioning for #flipbook IMG
. Additionally, we have to define relative positioning for the wrapper div (#flipbook
), so that the images position themselves within it and not a higher level wrapper.
As a side-product of this positioning, we need to set a height and width for both #flipbook
and #flipbook IMG
. This is due to the absolute positioning, which doesn’t force the wrapper to stretch to the height of the images.
The other main CSS concept here is that we are scrolling between images using z-index, which is the reason for the .active and .last-active classes. The opacity value is then used in conjunction with these z-indexes to allow the fade effect to run smoothly. If you are not using this effect, feel free to remove the opacity values so that the CSS validates. Just replace opacity: 0.0
with display: none
and opacity: 1.0
with display: inline
.
Finally, if you are using a different piece of markup than images for the flipbook, you should replace or mirror every IMG in flipbook.css with DIV or whichever tag you are using.
This looks like is something that I have been looking for the time to develop for a few years now. However, I have been trying to use your package with the ajax option and getting absolutely nowhere. I keep getting the error
“Flipbook.ajaxPhp is not a function” at the line in example.html where I placed “Flipbook.ajaxPhp( ‘flipbook.php’ );”
This seems to indicate that jQuery is calling my anonymous function before flipbook.js has created the Flipbook object. However, my grasp of jquery concepts is a bit shaky, so I wouldn’t take my analysis too seriously.
I am using your code without modifications – my images are in the directory /images/flipbook (relative to the domain root – actually it is a subdomain) and I placed the code for using the ajax option just above the flipbook div in the body of example.html as I thought I was supposed to.
What might I be doing wrong? You can see what I’ve done at
http://flips.dascher.net/example.html
thanks.
Hi David,
Totally my fault, I messed up the syntax in the example. Try this: Flipbook.ajaxPhp = ‘flipbook.php’;
Sorry about that and thanks for pointing it out,
Jon
Thanks for the great plugin. It works great for me except that I really need the images to display only once each. Can you please tell me how this can be done, it would be a great help.
Cheers
Paul
Hi Jon,
I was looking for just such a widget! Thanks so much. I was easily able to get it up-and-running using the image array approach. However, I’m having problems pulling the images from a directory with AJAX. Maybe I’m not understanding what Flipbook.ajaxPhp and Flipbook.init( ‘/images/flipbook’ ) represent. I assume that the latter represents the path to the images. I notice that you have an absolute path here. Can a relative path be used? If so, it is relative to what exactly? If not, i.e., we must use an absolute path, how would that work on a virtual or hosted website?
Thanks for any suggestions,
-Mark
great, very useful. butt do one can stop the flip after the last image?
Any way to pull at random from the array instead of sequential?
Hello,
We publish an iPad based magazine and it would seem your script is damn near perfect to create the “motion covers” that we’ve been looking to do.. (ie: http://www.youtube.com/watch?v=CI_W4uZ3C8U )
1. The only problem is that the script must be self contained. Is it possible to do this with “flipbook.html” as opposed to “flipbook.php”.
2. Is there a way to stop the animation after the last frame has played?
Great plugin, but we’re having trouble in I.E. 7 using PNG files. You can see all images in the background as it animates.
Very useful!
I have tried to enhance the plugin by allowing the HTML page to supply the flipbook.js with an extra parameter called lastImage. The call in the of the HTML page is then like this:
$(function() {
Flipbook.frameRate = 15;
Flipbook.lastImage = ‘countdown_136.png’; /* Added by Willem */
Flipbook.init( [‘./images/countdown_130.png’, ‘./images/countdown_131.png’, ‘./images/countdown_132.png’, ‘./images/countdown_133.png’, ‘./images/countdown_134.png’, ‘./images/countdown_135.png’, ‘./images/countdown_136.png’]);
});
Now within the flipbook.js I have added this lastImage parameter as well, like so:
….
var Flipbook = {
obj : {},
frameRate : 8,
lastImage : ‘lastImage.png’, /* Added by Willem */
fadeSpeed : 0,
ajaxPhp : ‘flipbook.php’,
frames : $(”),
imageCount : 0,
loadedImages : 0,
isBuilt : 0,
loadingAjax : 0,
….
All that is required now is that the code inside the slideSwitch : function() has an if / else statement that checks to see if the active image is equal to lastImage. If no, it should keep flipping. If yes, it should stop flipping. Like so:
…
if (XXX == lastImage) {
// reached last image, stop flipping
break;
}
else {
Flipbook.obj.active = $next;
}
…
However, I can not yet figure out what I should type instead of the XXX. How do I get the name of the active image to compare it to the lastImage parameter??? Any help much appreciated !!
For last two days I am trying to customise this jquerry plugin but getting nowhere. i just need to add play, pause, stop and replay functions in this plugin. Can you tell me what is the path I need to take in order to achieve this.
Great script!
I am looking for a way to pause the animation start until the animation is in the window view.